Arduino Development on OpenBSD
Back in 2017, I bought an Arduboy, a fun little Arduino development system which integrates an ATmega32U4 8-bit CPU, 32 KB of flash storage, 2 KB of RAM, a 128x64 pixel OLED display, some buttons, a speaker, and a battery in a Gameboy-like package.
OpenBSD had an
old Arduino package
available without the
Arduino IDE, and it instead included
a custom
Makefile
for end-users to build off of for compiling projects.
But it was all pretty old and crufty and kind of sucked the fun out of tinkering
with a new piece of hardware.
I was eventually able to compile and upload some test code from OpenBSD but I couldn't easily link in the Arduboy2 library which is something the Arduino IDE makes very easy.
Arduino-Makefile
In 2018, I found
Arduino-Makefile
which was like our custom Makefile
but well-maintained and much easier to use.
For my Arduboy game, it required a
Makefile
as simple as:
BOARD_TAG = leonardo
include /usr/local/share/arduino-makefile/Arduino.mk
I created OpenBSD ports of Arduino-Makefile and the Arduboy2 library, and updated the Arduino port to a newer version. With that, I was able to rapidly work on my 1010 game and finish it.
Adafruit Metro
Last week I bought an
Adafruit Metro
and their
RA8875 LCD-interface
board and wanted to quickly get them displaying something on an LCD via my
OpenBSD laptop.
When plugged into a USB port, the Metro attaches as a uslcom
device and powers
up:
uslcom0 at uhub0 port 4 configuration 1 interface 0 "Silicon Labs CP2104 USB to UART Bridge Controller" rev 2.00/1.00 addr 6
ucom0 at uslcom0 portno 0
I installed the arduino-makefile
package which brings in the
arduino
and avrdude
packages:
# pkg_add arduino-makefile
Adafruit provides a simple buildtest.ino
file for the RA8875 in their
library,
which I fetched and then made a simple Makefile
to build it:
$ mkdir buildtest && cd buildtest
$ ftp https://raw.githubusercontent.com/adafruit/Adafruit_RA8875/master/examples/buildtest/buildtest.ino
$ cat > Makefile
BOARD_TAG = metro
include /usr/local/share/arduino-makefile/Arduino.mk
^D
$ gmake
This initially failed to build because metro
was not in the Arduino's
boards.txt
file being an Adafruit product.
I
updated
the OpenBSD arduino
package to include
Adafruit's boards.txt
which has a definition for metro
.
Next, I needed Adafruit's
RA8875 library
which itself needed their
GFX library.
To use a 3rd party Arduino library, just check it out from GitHub or wherever,
and copy the whole thing to /usr/local/share/arduino/libraries
where you'll
see other directories like EEPROM
and SPI
.
Creating OpenBSD ports/packages of these is not necessary to get working quickly,
but it's very easy to do and helps out anyone else wanting to use them.
I did so and
imported
them
so that one can just pkg_add arduino-adafruit-ra8875
.
Once those libraries were installed, I added them to the Makefile
:
ARDUINO_LIBS = EEPROM SPI Adafruit_GFX Adafruit_RA8875
Normally Arduino-Makefile can find and bring in libraries automatically but if it
can't (such as when a library is a dependency of another library), you may need
to explicitly list them in an ARDUINO_LIBS
variable.
After a successful compilation with gmake
, the build-metro/buildtest.ino
file
can be uploaded to the Metro board with gmake upload
.
This single step calls ard-reset-arduino
(from Arduino-Makefile) to send the
magic sequence to the device (/dev/ttyU0
by default) to put it into its
bootloader mode, and then uses avrdude
to upload the binary code and run it.
$ gmake upload
[...]
mkdir -p build-metro
gmake reset
gmake[1]: Entering directory '/home/jcs/code/buildtest'
/usr/local/bin/ard-reset-arduino /dev/ttyU0
gmake[1]: Leaving directory '/home/jcs/code/buildtest'
gmake do_upload
gmake[1]: Entering directory '/home/jcs/code/buildtest'
/usr/local/bin/avrdude -q -V -p atmega328p -D -c arduino -b 115200 \
-P /dev/ttyU0 -U flash:w:build-metro/buildtest.hex:i
avrdude: AVR device initialized and ready to accept instructions
avrdude: Device signature = 0x1e950f
avrdude: reading input file "build-metro/lcdtest.hex"
avrdude: writing flash (13340 bytes):
avrdude: 13340 bytes of flash written
avrdude: safemode: Fuses OK
avrdude done. Thank you.
gmake[1]: Leaving directory '/home/jcs/code/buildtest'
$
With that, I was seeing some nifty graphics on my new LCD hooked up to my Metro board.
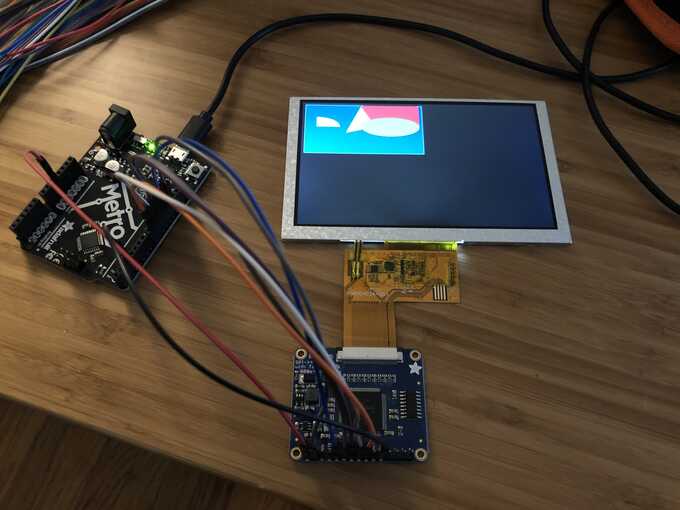
The buildtest
program sends some debugging information over its serial port
while it runs:
void setup()
{
Serial.begin(9600);
Serial.println("RA8875 start");
[...]
This data can be monitored with cu
:
$ sudo cu -l /dev/cuaU0 -s 9600
Password:
Connected to /dev/cuaU0 (speed 9600)
RA8875 start
Found RA8875
Status: 0
Waiting for touch events ...